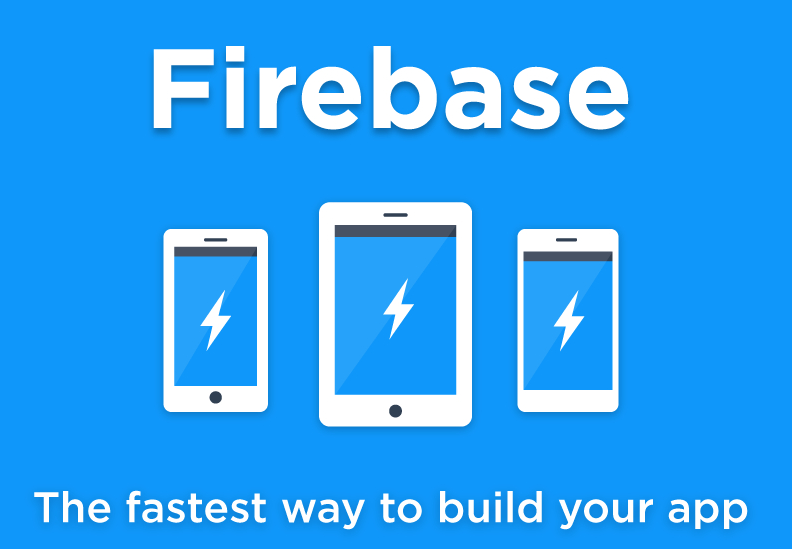
Google推送firebase PHP代码
代码如下:
<?php
/**
* google推送
* Firebase -> Project Overview -> Project settings -> Cloud Messaging -> Server key
*
* @author kevinxie
* @date 2020/05/09
*/
class Lib_Googlepush
{
protected static $_instance = array();
private $pushUrl = 'https://fcm.googleapis.com/fcm/send';
public function __construct()
{
}
/**
* 对像生产器
*
* @return object
*/
public static function factory()
{
if ( !isset( self::$_instance[__METHOD__] ) || !is_object( self::$_instance[__METHOD__] ) )
{
self::$_instance[__METHOD__] = new self();
}
return self::$_instance[__METHOD__];
}
/**
* 谷歌推送-单条
*
* @param string $registrationId 客户端获取的用户token
* @param array $arrContent 推送内容 array('title'=>string, 'body'=>string, 'data'=>array(key=>value))
* @return bool
*/
public function pushOne( $registrationId, $arrContent)
{
if( $arrContent['title'] == '' || $arrContent['body'] == '')
{
return false;
}
$fields = array();
$fields['to'] = $registrationId;
$fields['notification'] = array( "title"=>$arrContent['title'], 'body'=>$arrContent['body']);
if( is_array( $arrContent['data']))
{
$fields['data'] = $arrContent['data'];
}
$configGoogle['serverKey'] = 'google serverKey';
if( $configGoogle['serverKey'] == '')
{
return false;
}
$headers = array(
'Authorization:key='.$configGoogle['serverKey'],
'Content-Type:application/json',
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $this->pushUrl);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($fields));
$result = curl_exec($ch);
if ($result === FALSE)
{
return false;
}
curl_close($ch);
$arrResult = json_decode( $result, true);
if( $arrResult['success'] == 1)
{
return true;
}
else
{
return false;
}
}
/**
* 谷歌推送-多条
*
* @param array $arrParam array('registration_ids'=>array(token,token...), 'title'=>string, 'body'=>string, 'data'=>array()))
* @return bool
*/
public function pushMany( $arrParam)
{
if( ! is_array( $arrParam))
{
return false;
}
$fields = array();
$count = count($arrParam['registration_ids']);
if( $count == 0 || $count > 1000)
{
return false;
}
$fields['registration_ids'] = $arrParam['registration_ids'];
$fields['notification'] = array( "title"=>$arrParam['title'], 'body'=>$arrParam['body']);
if( is_array( $arrParam['data']))
{
$fields['data'] = $arrParam['data'];
}
$configGoogle['serverKey'] = 'google serverKey';
if( $configGoogle['serverKey'] == '')
{
return false;
}
$headers = array(
'Authorization:key='.$configGoogle['serverKey'],
'Content-Type:application/json',
);
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $this->pushUrl);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($fields));
$result = curl_exec($ch);
if ($result === FALSE)
{
return false;
}
curl_close($ch);
$arrResult = json_decode( $result, true);
if( $arrResult['success'] >= 1)
{
return true;
}
else
{
return false;
}
}
}
// end class Lib_Googlepush
?>
单条推送调用方法:
$registrationId = 'google token';
$arrContent = array('title'=>'title x1', 'body'=>'body x1', 'data'=>array('参数名'=>'值'));
Lib_Googlepush::factory()->pushOne( $registrationId, $arrContent);
批量推送调用方法:
$arrSendTokens = ['google token 1','google token 2',....];
$arrParam = [];
$arrParam['registration_ids'] = $arrSendTokens;
$arrParam['title'] = 'title x2';
$arrParam['body'] = 'title x2';
$arrParam['data'] = array('参数名'=>'值');
Lib_Googlepush::factory()->pushMany( $arrParam);
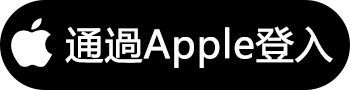
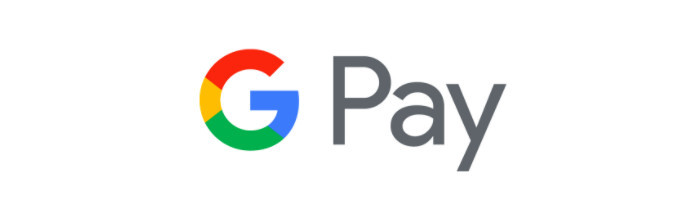